This guide takes you through the steps to install and set up the new HC-SR04 distance measuring sensor Python Library. First, we connect the ultrasonic module to the Raspberry Pi GPIO pins. Then, we use pip to install the Python module. Followed by, a walkthrough of the ultrasonic Python module features.
There are plenty of guides around that explain how to connect the sensor module to the Raspberry Pi GPIO. But no real software solution is offered. Therefore, this guide will focus mainly on the software.
HC-SR04 Distance Measuring Transducer
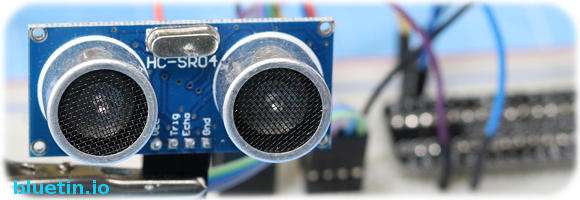
This sensor uses sound waves to provide a means to measure the distance between the sensor and an object. It is not the most accurate distance sensor available, but many projects do not need pinpoint accuracy. After a quick look at Banggood website, you can get five HC-SR04 sensors for 5.07 GBP (6.86 USD). And while the sensor is not the most compact, its low price means a robot vehicle can have a full sensor kit fitted very cheaply.
The Python Library
I wrote the Python Library to support this sensor in projects I create from now on. The Python library adds enough flexibility to allow adjustments for more accurate distance measuring reads. Furthermore, supporting this sensor through a Python package adds the convenience for a quick install across many Raspberry Pi boards. Check below for details on how to get this library and how to use it.
HC-SR04 Connection and Library Setup
Here we begin the setup process of the ultrasonic sensor. Some knowledge of how to set up a Raspberry Pi and run Python code is assumed.
HC-SR04 Connection
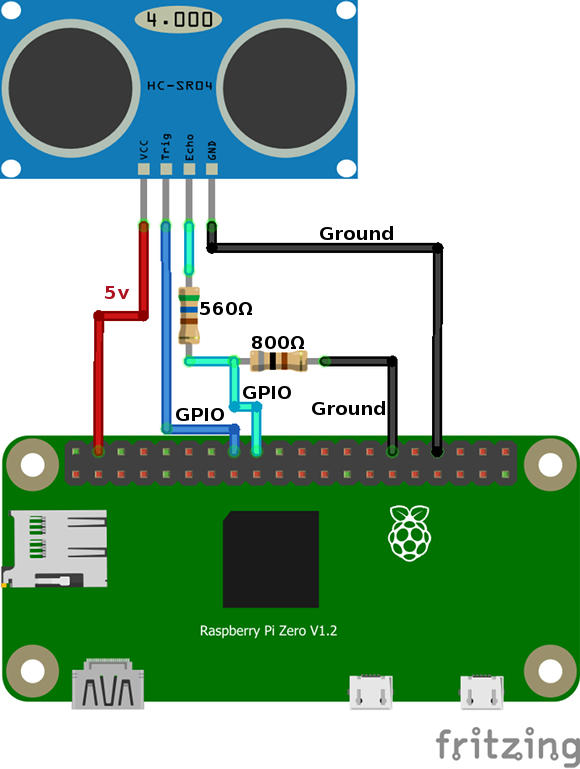
I set up the circuit on a breadboard which made it easier to connect a resistor voltage divider. You can plug the SH-SR04 sensor into the breadboard directly or by attaching a ribbon cable. Additionally, I use a T-Cobbler that allows me to connect the Raspberry Pi GPIO pins to the breadboard. The Raspberry Pi will power the circuit from one of its 5v pins.
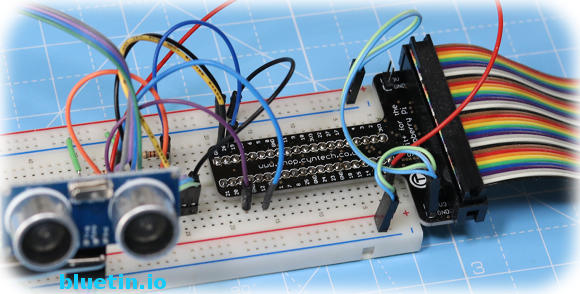
You can use any free GPIO pins for the distance measuring sensor Trigger and Echo pins. The SH-SR04 Python library uses BCM – Broadcom pin numbers for pin assignments.
The resistor voltage divider is necessary to reduce the 5v output signal of the sensor to less than 3.3v. The resistor values in the circuit illustration above decrease the voltage to just less than 3v. This lower voltage is still enough for the Raspberry Pi to read a high signal. Exposing the Raspberry Pi GPIO pin to more than 3.3v may cause permanent damage to the board.
Furthermore, for non-experts, you can use a voltage divider calculator on the internet to find suitable resistor values. If you have a large resistor starters kit, there will be no problems finding suitable resistors.
HC-SR04 Python Library Setup – Bluetin_Echo
The HC-SR04 distance measuring transducer is not a complicated sensor to interface with code. However, packaging this sensor into a library allows useful calibration and output methods to be available as standard. Furthermore, the library also makes it easier to add multiple sensors and avoid cluttering up the main program code. The library is called Bluetin_Echo and can be installed using the PIP installer.
Bluetin_Echo Python Package Features:
- Can be installed using the Python PIP installer.
- You can choose to return results in either MM, CM and Inch.
- Using a library property, you can change the default speed of sound value at any time.
- You can change the HC-SR04 rest period between reads.
- Sensor calibration can be made from a known distance between sensor and object.
- The library can return an average result from any number of sensor reads.
- If an echo is not detected, a timeout will return 0 as a result. So this timeout will avoid a program stall.
Installing Bluetin_Echo for HC-SR04
To install the Bluetin_Echo library for the HC-SR04 sensor, open a Terminal on the Raspberry Pi, then execute the command below. However, remember to open an Isolated Python Environment if you have that setup. There is more about Isolated Python Environments here.
pip install Bluetin_Echo
If you have the Bluetin_Echo library on the Raspberry Pi already, try upgrading with the following command.
pip install --upgrade Bluetin_Echo
Example Python Code for HC-SR04 Sensor
Import the Bluetin_Echo library into your program:
from Bluetin_Echo import Echo
Define the Raspberry Pi GPIO pins that are connected to the Ultrasonic HC-SR04 Distance Measuring Sensor:
TRIGGER_PIN = 16 ECHO_PIN = 12
Initialise the distance sensor with the pins defined above. Optionally, you can change the default speed of sound value (343) to a calibrated value:
speed_of_sound = 340 echo = Echo(TRIGGER_PIN, ECHO_PIN, speed_of_sound)
Get a distance measurement. We can choose just to take one measurement sample or get an average of more than one sample.
Get distance measurement without average result.
In this case, the sensor will take just one measurement. However, this is a non-blocking call and will return the last sensor measurement if the HC-SR04 sensor has not completed the rest period. Therefore, calling this method back to back will likely return the same result multiple times until the sensor rest period has expired.
So, this feature is useful if the main program is looping much faster than the sensor rest period. Therefore, the main Python program will not be interrupted by extra delays and still get valid sensor results. However, the downside is that single shot distance measuring may not be as accurate as averaging multiple readings.
# Units include mm, cm and inch result = echo.read('cm') # Or result = echo.read('cm', 1) # Print result. print(result, 'cm')
Return an average of more than one distance measurement
Requesting an average of more than one distance measurement will likely improve accuracy and consistency. Furthermore, this method will allow the distance sensor to rest between each measure. The rest period is user adjustable and 0.06 second appears to be the sweet spot.
# Number of distance measurements to average. samples = 5 # Get an average of distance measurements. result = echo.read('cm', samples) # Print result. print(result, 'cm')
GPIO Reset
Before the program closes, do a Raspberry Pi GPIO cleanup. However, you only need to add this line once at the end of the program for all GPIO instances.
# GPIO cleanup echo.stop()
Example Python Programs for HC-SR04 Sensor
Accuracy
The sensor echo timings are going to fluctuate under the Raspbian operating system. So, by calibrating the sensor against a large flat surface, you will have some idea of the extent of the fluctuations. Then, the fluctuations can be compensated for in the final code of the project.
Example code
Here are some example programs you can try out. You can find these programs and more on the following GitHub page.
- GitHub Page – Bluetin_Python_Echo – Link.
Simplest Program for Distance Measuring:
"""File: echo_simple_once.py""" # Import necessary libraries. from Bluetin_Echo import Echo # Define GPIO pin constants. TRIGGER_PIN = 16 ECHO_PIN = 12 # Initialise Sensor with pins, speed of sound. speed_of_sound = 315 echo = Echo(TRIGGER_PIN, ECHO_PIN, speed_of_sound) # Measure Distance 5 times, return average. samples = 5 result = echo.read('cm', samples) # Print result. print(result, 'cm') # Reset GPIO Pins. echo.stop()
Example for Taking Multiple Distance Measurements:
"""File: echo_loop.py""" # Import necessary libraries. from Bluetin_Echo import Echo # Define GPIO pin constants. TRIGGER_PIN = 16 ECHO_PIN = 12 # Initialise Sensor with pins, speed of sound. speed_of_sound = 315 echo = Echo(TRIGGER_PIN, ECHO_PIN, speed_of_sound) # Measure Distance 5 times, return average. samples = 5 # Take multiple measurements. for counter in range(0, 10): result = echo.read('cm', samples) # Print result. print(result, 'cm') # Reset GPIO Pins. echo.stop()
Loop Through Multiple Sensors
"""File: echo_multi_sensor.py""" # Import necessary libraries. from time import sleep from Bluetin_Echo import Echo # Define pin constants TRIGGER_PIN_1 = 16 ECHO_PIN_1 = 12 TRIGGER_PIN_2 = 26 ECHO_PIN_2 = 19 # Initialise two sensors. echo = [Echo(TRIGGER_PIN_1, ECHO_PIN_1) , Echo(TRIGGER_PIN_2, ECHO_PIN_2)] def main(): sleep(0.1) for counter in range(1, 6): for counter2 in range(0, len(echo)): result = echo[counter2].read('cm', 3) print('Sensor {} - {} cm'.format(counter2, round(result,2))) echo[0].stop() if __name__ == '__main__': main()
The output as follows:
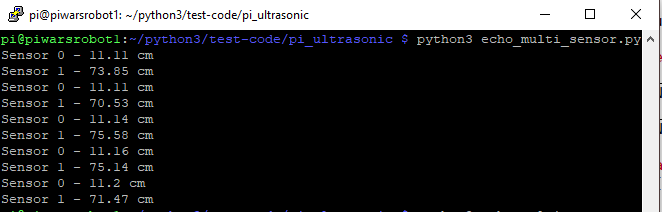
Other Properties and Methods for HC-SR04 Sensor
The speed property allows you to set the speed of sound while the program is running. The current default is 343 meters per second.
# Set and get speed of sound echo.speed = 320 print(echo.speed)
It is important to set enough delay between sensor echo reads for returning consistent values. The default for this property is 0.06 second.
# Set and get sensor rest period echo.rest = 0.07 print(echo.rest)
Distance Sensor Calibration
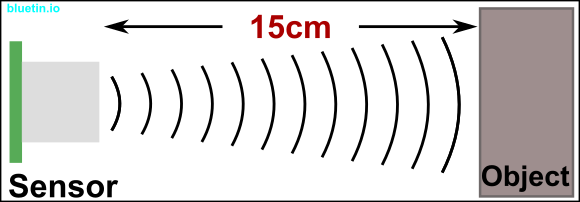
This method will help you calibrate the distance sensor. Measure the distance between sensor and object. Then use this measurement in the calibrate method to set a new speed of sound. The speed of sound value is also returned from this method.
This calibration feature is useful for automated calibration to a known distance. For instance, you might have the sensor on a pan and tilt platform where you can point the sensor to a fixed object for calibration.
sleep(0.1) #Calibrate sensor to a known distance. distance = 15 unit = 'cm' samples = 20 value = echo.calibrate(distance, unit, samples) print('Calibrated to {} meters per second'.format(value))
Buying Featured Items
DISCLAIMER: This feature may contain affiliate links, which means that if you click on one of the product links, I’ll receive a small commission. This commission helps support the website and allows me to continue to make features like this. Thank you for the support!
Banggood:
- 5Pcs Geekcreit® Ultrasonic Module HC-SR04 Distance Measuring Ranging Transducer Sensor DC 5V 2-450cm – Link.
UK Searches:
UK Amazon:
- Raspberry Pi – Search.
- MicroSD Card – Search.
- Raspberry Pi Compatible Wi-Fi Dongle – Search.
- Raspberry Pi Camera – Search.
US Searches:
US Amazon:
- Raspberry Pi – Search.
- MicroSD Card – Search.
- Raspberry Pi Compatible Wi-Fi Dongle – Search.
- Raspberry Pi Camera – Search.
On Closing
I hope you find this article useful – Ultrasonic HC-SR04 Sensor Python Library for Raspberry Pi GPIO, please like and share.