Using an isolated Python Environment for developing code is a practice I should be employing. So this article is a guide to setting up and using a virtual environment for Python projects.
Creating an isolated Python environment with a tool called virtualenv is a way to separate different Python projects. So instead of having every Python project sharing the same dependencies, we can isolate projects with its own specific dependencies. As a result we avoid breaking some Python projects through global site-packages updates.
Beginners
For those who are new to Python, dependencies are basically packages that contain a collection of modules. Some modules are already included with Python, but it is likely that you will soon start installing your own modules. Modules may also be referred to as libraries. Popular libraries you might include in your Python project, for example, are:
- Requests -The http library by Kenneth Reitz.
- NumPy – It provides some advance maths functionalities.
- SciPy – A library of algorithms and mathematical tools.
- Pygame – A game development library.
Anybody beginning to use Python will benefit from creating isolated Python environments. Thus this will keep the global site-packages neat and tidy.
Isolated Python Environment
Problem
Isolated Python environments might not seem like a big deal at first. But when you come to install libraries to support OpenCV for instance, you might want to be doing everything you can to protect it. After all, you might have spent hours pacing up and down watching the libraries compile.
If you work on many different projects in the usual Python environment, over time, you are likely to install and update many packages. Consequently, this will cause the global site-packages directory to change constantly. Continuing to install and update Python packages might eventually cause some Python projects to stop working – as well as the OpenCV libraries you might have installed.
Solution
Basically, Isolated Python Environment allow me to develop and run Python projects in separate clean environments. I can install Python modules specific to my project in its own isolated environment. As a result, my project will be protected from global site-packages updates.
A newly created Python Environment will not include packages from the site-packages directory. Therefore, pip package manager will be used to add the packages the project requires.
Set-up Isolated Python Environment
You will want to login to the platform you are working with. The following commands will be entered in the terminal. Commands are for both Python 2 and Python 3. However, do not enter the comment lines that begin with the hash (#) character.
Now it is time to install the isolated Python environment package. For my purpose, I’m using Raspberry PI with Raspian Stretch Lite installed. If I remember correctly, only the Raspbian desktop versions have pip installed. So install Python pip, if you don’t have it installed already. To test for pip you can try the following:
pip -h pip3 -h
You can install pip for each version of Python or you can just install the one you prefer:
sudo apt-get install python-pip sudo apt-get install python3-pip
Next we install isolated Python environment packages. Then we clear the pip cache:
sudo pip install virtualenv virtualenvwrapper sudo pip3 install virtualenv virtualenvwrapper sudo rm -rf ~/.cache/pip # Careful with this command.
We add some lines to the profile file so that virtualenvwrapper can be set-up in each new terminal login. We first add a description line, then we tell virtualenvwrapper where we want the virtual folders to be stored. Then the virtualenvwrapper installation supplied script is added.
echo -e "\n# virtualenv and virtualenvwrapper" >> ~/.profile echo "export WORKON_HOME=$HOME/.virtualenvs" >> ~/.profile echo "source /usr/local/bin/virtualenvwrapper.sh" >> ~/.profile
Optionally, append further lines to the profile file so that we can get a reminder message after terminal login. The reminder message tell us how to create or open a project virtual environment.
echo “echo \”Virtual Python Environment\”” >> ~/.profile echo “echo \”$ mkvirtualenv project_name -p python3\”” >> ~/.profile echo “echo \”$ workon project_name\”” >> ~/.profile
Create Isolated Python Environment
If you are continuing from above, updating profile file, you will need to logout, login. Else you can run the following command to reload the profile set-up.
source ~/.profile
Finally, we create our first Python environment. You could name the new environment that relates it to a project it belongs to. The project robot_cv is just a random project name. The purpose of the following command is only to create virtual environments. We use a different command, later in the next section, to enter virtual environments we create.
mkvirtualenv robot_cv -p python2 mkvirtualenv robot_cv -p python3
So there you have it. First isolated Python environment created.

The terminal command prompt above show that the virtual environment is active. You will see which environment we are currently in by looking at the project name in brackets.
deactivate
Thus we return to the normal command prompt by using the deactivate command. This will take us out of the virtual environment.
Using Isolated Python Environment
Brief
All the virtual environment commands we use are provided by the virtualenv wrapper. This adds more flexibility than had we only installed the virtualenv package. So when you come to lookup commands to use the virtual environment, you need virtualenvwrapper command reference.
While you are in the virtual environment you will still be able to navigate around your home user directory as normal. So you can navigate to your python project files and run them as you did before.
However, you need to remember, your python project will not have access to global site-packages while in the virtual environment. But you can still install packages that your project needs by using the pip install command.
Useful Commands
Create A New Virtual Environment
mkvirtualenv robot_cv -p python3
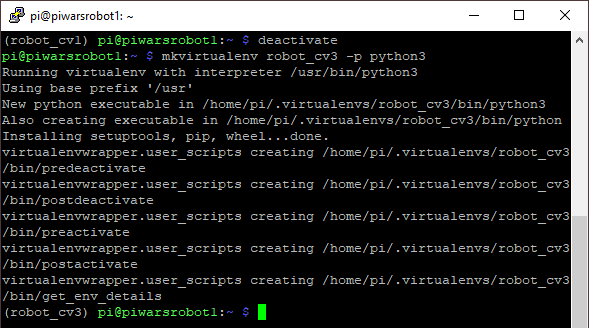
Deactivate The Current Evironment
deactivate

Delete A Virtual Environment
rmvirtualenv robot_cv3
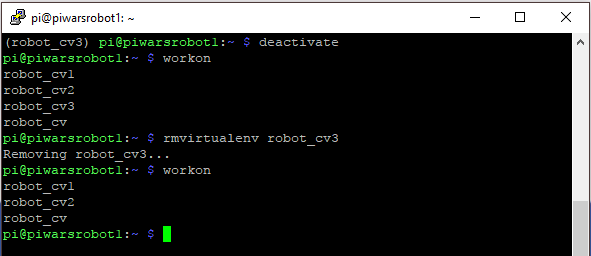
Load A Virtual Environment To Work In
At the terminal command prompt, to list all the virtual environments you have created, run the following command:
workon
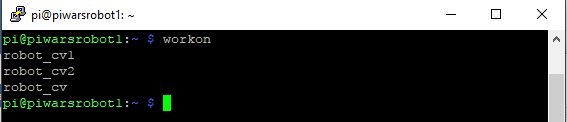
To jump in a virtual environment we just select one we have created before:
# robot_cv is the name of the virtual environment I created earlier. workon robot_cv
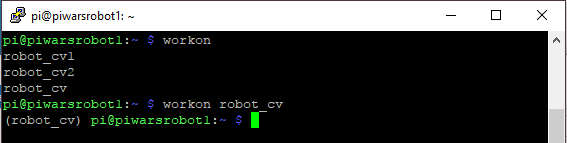
So now that your in the virtual environment, you can perform some of the following commands.
Run A Python Program
python3 python3/helloworld/helloworld.py

Switch Virtual Environments
workon robot_cv2

You will notice the change by checking the name within the brackets on the command prompt.
List Python Packages available In Current Virtual Environment
pip3 freeze --all
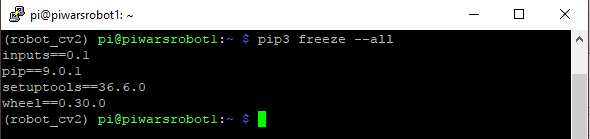
Python Package List output To Text File
pip3 freeze --all > robot_cv2_req.txt

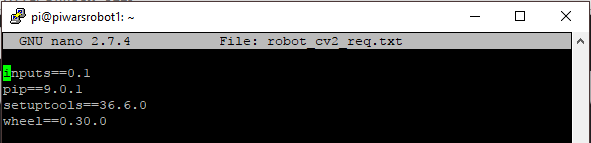
Install Packages From List
pip3 install -r robot_cv2_req.txt
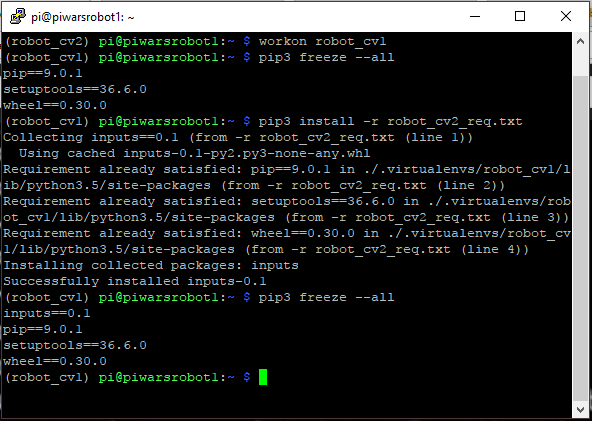
Virtualenv Wrapper Command List
- workon
- deactivate
- lsvirtualenv
- showvirtualenv
- mkvirtualenv
- rmvirtualenv
- allvirtualenv
- mktmpenv
- add2virtualenv
- cdsitepackages
- lssitepackages
- cdvirtualenv
- mkproject
- setvirtualenvproject
- cdproject
- wipeenv
Links
- virtualenvwrapper Command Reference – Link.
- pip Reference Guide – Link.
- virtualenv Reference Guide – Link.
Related Articles
How To Start Raspberry Pi Programming – Link.
Buying Featured Items
The purchase price is going to vary greatly depending on how quickly you want the items. Therefore shop around checking out Amazon, Ebay, Adafruit and local electronic stores.
UK Searches:
UK Amazon:
US Searches:
US Amazon:
On Closing
I hope you fined this article useful – Raspberry Pi Controller For Robot Control Review, please like and share.